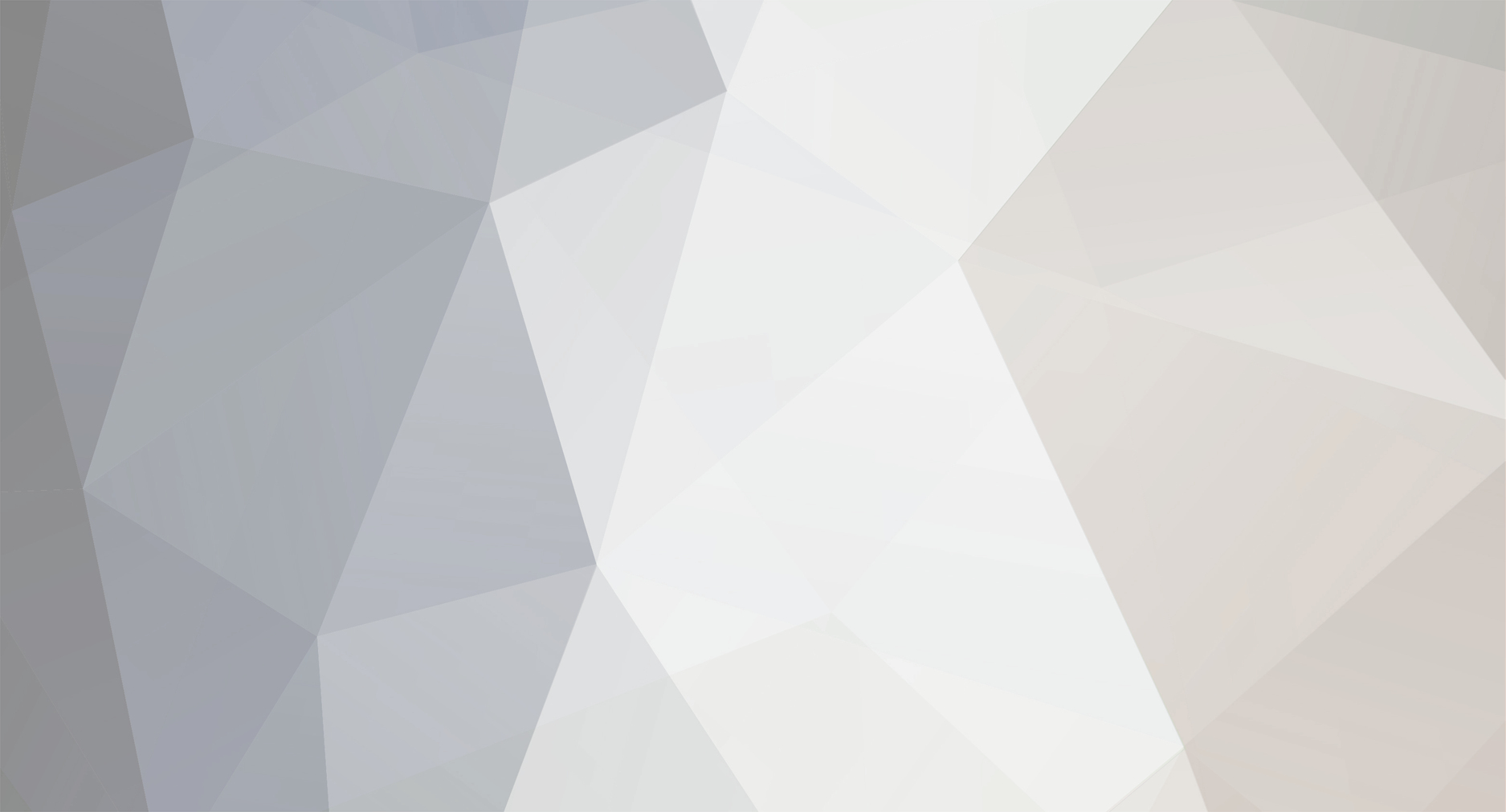
CmP
Contributor-
Posts
668 -
Joined
-
Last visited
-
Days Won
49
Content Type
Profiles
Forums
Downloads
Gallery
Everything posted by CmP
-
Is this opinion based on facts or your subjective preferences? Almost all claims don't correspond to reality. 1. "ancient methods of patching". Restoring saved list has been there before scripts, so it's clearly the other way around. 2. "loadlists are faster". Based on what? Where are the results of performance tests? What's obvious without tests is that both methods are "instant" for editing hundreds or even few thousands values. 3. "loadlists are easier to update". Nothing prevents one from writing script in a way to be as easy updatable as possible, for example, with configuration table. And format of saved lists is far from being comfortable to work with manually. 4. "loadlists take less code". They do, at cost of losing all flexibility. How to restore only some of patched values with saved lists? To keep saved list for each desired state of values? What if there are tens of such states? And for some reason one of the most obvious disadvantages of saved lists isn't mentioned. It is required to (at least temporary) have a file for each one. So instead of directly patching values you suggest to create a file with saved list data and load it only to accomplish the same. If that isn't highly redundant approach, then I don't know what is.
-
Rewrite of the loop with inner loops with comments to main fixed issues (one mistake and one statement misplacement): local executable = {} for i = 1, #exe do local stringBytes = {} local startAddress = strPointer[i].value - 1 for j = 1, 150 do stringBytes[j] = {address = startAddress + j, flags = gg.TYPE_BYTE} end stringBytes = gg.getValues(stringBytes) local stringChars = {} for index, byte in ipairs(stringBytes) do local value = byte.value if value == 0 then -- comparison to string "0" is a mistake since value field is a number break end stringChars[index] = string.char(value & 0xFF) end local str = table.concat(stringChars) -- get final string only once after construction of table with characters is finished executable[i] = {address = exe[i].address, flags = gg.TYPE_DWORD, name = str} end gg.addListItems(executable)
-
Speedhack diagnostics: Lib6.so not found in some games?
CmP replied to bluechipps's question in Help
Before you proceed to try different or modified apks of the games, consider checking structure of installed files of games for which SH loads and for which doesn't to see, whether there are any patterns there. Root folder to start checking and comparing from is game's folder (or rather sub-folder that includes package name) in /data/app. -
Speedhack diagnostics: Lib6.so not found in some games?
CmP replied to bluechipps's question in Help
I confirm this observation, "Stop, but not a breakpoint" seems to be unintended result. The logs also contain some details about what goes wrong when this happens. The first difference of interest is on the line that starts with "breakpoint:". "WSTOPSIG(5)" in logs with loaded SH means that process has been stopped because it received SIGTRAP signal, i.e. a breakpoint has been encountered. "WSTOPSIG(11)" in logs with failure, on the other hand, means that process has been stopped because it received SIGSEGV signal which is caused by invalid memory access. The second difference of interest, that allows to understand what caused invalid memory access, is on the following line that starts with "aarch64:" and contains information about values in registers. In logs with loaded SH value in "pc" (program counter) register is some existing address in process memory, for example, 0x703142802c from "loaded_shatteredpixel.txt". In logs with failure it is different, value in "pc" register is not an existing address in process memory, since it is clearly too small for that (for example, 0x8be3f0 from "failed_dreadrune.txt"). One possible interpretation of the differences described above is that in cases of failure to load SH something (maybe injected code) for some reason causes jump to invalid address that, as has been mentioned, causes SIGSEGV. Unfortunately, the logs by themselves don't allow to investigate further, that would most likely require using a debugger and would be significantly more complicated than analysis of logs. -
String contents doesn't matter. If two strings are compared, they are compared accordingly. It's a simple system. It gets more complicated (and confusing) when variety of implicit conversions may be involved, like for example in JavaScript.
-
For "equal" it doesn't matter much, since comparison of strings will work as well. For "greater than" and "less than" it is correct solution and string comparison is not. String comparison works by comparing strings character-by-character in alphabetical order, so it's not applicable for comparing strings that represent versions.
-
There is built-in way to ensure that version is not older than specified one, by using "gg.require" function. For example: gg.require("101.1") One other important note is NOT to compare version strings using operators ">" and "<" like it's done in some answers in this topic, because it won't work as expected in all cases. The condition "99.0" > "101.0" evaluates to true, but version 99.0 is not greater than 101.0. That's why to compare versions using by "greater than" or "less than" conditions "gg.VERSION_INT" constant that MAARS has mentioned in his answer should be used instead.
-
The main issue is with the approach itself. Processing all results/values at once isn't going to work when there are millions of them. There is simply not enough memory that is available to Java part of the application neither to load millions of results at once nor to build a table in Lua script that represents millions of values. The solution is to process results/values in parts. Reasonable choice for part size is around 100k, since in practice GG can load 100k results fine in most cases. Another issue is related to efficiency as MAARS also mentioned above. First thing to avoid is using "gg.loadResults" function when it's not necessary. If it's applicable, use searches to get all results for processing, then process them in parts by getting results and removing them after the part is processed. Alternatively build a table with part of values to process, but then don't use results list, proceed to getting/setting values directly using corresponding API functions, then repeat for remaining parts.
-
There is "one small issue" with this example. It implies that GG daemon would attach to itself. Not only it can't work like that, GG also doesn't show it's own daemon in process list (for the aforementioned reason). You clearly confused something in this example, but instead of admitting it, you have resorted to suggesting that critique of your example stems from "lack of knowledge". Such desperate attempts to "never make mistakes" is a sign of arrogance and an obstacle that prevents learning from one's own mistakes.
-
This example doesn't make sense. Only memory of selected process can be modified. Scripts have no control over which process is selected. So the question remains, what does GG daemon have to do with this?
-
How to "hook the GG daemon" from script for GG? Or what is this supposed to mean? Where in a process that is selected in GG would there be anything related to GG daemon? As for executing arbitrary code, the approach from your previous sentence already allows that, but of course code can only be executed in context of the process that is selected in GG. So what does GG daemon have to do with this?
-
Most likely it was meant to configure GG to work with SELinux. Start screen, "Fix it" button, "Switch to work with SeLinux and restart the app" option. Reference: Android 11 My phone is rooted but game gaurdian not work properly I'm downloading latest version it's not fix (#axy2r12m)
-
Having password that is required to execute a script for GG is not a good idea in general, but having limited password (by expiration time, amount of uses or something else) is a faulty one, because the limit can't be enforced anyhow. If a script for GG can be executed once, this is already enough to preserve all of the required data to execute it again unlimited amount of times regardless of password. In other words, password for a script for GG can only work as initial protection: either user doesn't know the password and won't be able to execute the script at all or user knows the password and with certain preparations will have unlimited access to the script after executing it one time.
-
This is absolutely not the case. Here is an example of packet capture application for Android that can show users decrypted contents of HTTPS requests and responses from/to GG: https://play.google.com/store/apps/details?id=app.greyshirts.sslcapture Similarly, there are other applications for Android and a bunch of much more well-known ones for Windows, each of which has this capability.
-
There was a mistake in my last example. Edited the post with fixed version, so probably it should work as expected now.
-
If I understood correctly, it's because you also need to edit next instruction to "RET" for your patch to work as expected. The example above can be modified to do that: local libStartAddress = gg.getRangesList("libil2cpp.so")[2].start local targetAddress = libStartAddress + 0x14BB114 gg.setValues({ {address = targetAddress, flags = gg.TYPE_DWORD, value = "~A8 MOV W0, #999"}, {address = targetAddress + 4, flags = gg.TYPE_DWORD, value = "~A8 RET"} }) Edit: corrected mistake in previous version of the code
-
Yes, just use that edit string as "value" field. For example: local libStartAddress = gg.getRangesList("libil2cpp.so")[2].start local targetAddress = libStartAddress + 0x14BB114 gg.setValues({{address = targetAddress, flags = gg.TYPE_DWORD, value = "~A8 MOV W0, #999"}})
-
When GG reinstalls itself, version of the apk is set to a random one. That's where version "239.0" came from in your case.
- 1,992 replies
-
2
-
- GameGuardian APK
- Official Download
-
(and 1 more)
Tagged with:
-
In the description of the video there is the following: So at the very least author of the video has specified who and where originally posted the method. Of course it would be more reasonable to include link to this thread, but at least there is some mention at all.
-
help Lua Script: How to get utf-8 characters from memory and alert
CmP replied to dolphinorca's question in Help
The idea is to read enough bytes at once and then trim the string to first occurrence of quote character instead of reading one byte at a time until quote character is encountered. Generally, first approach should be better in terms of performance, because each read from memory takes significantly more time than several Lua operations, even if just one byte needs to be read. I thought to add 12 to get position of first byte of the answer that is the next one after last quote character in searched string. I don't immediately see why it doesn't work as expected, but it seems that there is a mistake somewhere and it's fixed by your correction to use "+ 11" instead of "+ 12".- 10 replies
-
help Lua Script: How to get utf-8 characters from memory and alert
CmP replied to dolphinorca's question in Help
You should also consider refactoring the code to make it simpler and cleaner. For example, your "MENU1" function can be refactored to something like the following: function MENU1() gg.setRanges(gg.REGION_ANONYMOUS) gg.clearResults() gg.searchNumber(':","answer":"', gg.TYPE_BYTE) local count = gg.getResultsCount() local results = gg.getResults(count) local answers = {} for i = 1, count, 12 do local address = results[i].address + 12 local maxLength = 1024 local answer = readBytesToString(address, maxLength) answer = answer:match('[^"]*') -- match part up to first quote character table.insert(answers, answer) end for number, answer in ipairs(answers) do print(string.format("Answer #%d:", number), answer) end gg.alert("Answer See [ON]") end Let me know, if the code above works for you.- 10 replies
-
help Lua Script: How to get utf-8 characters from memory and alert
CmP replied to dolphinorca's question in Help
To output a string Lua implementation in GG treats it as UTF-8 (by themselves Lua strings are just byte arrays), so reading bytes from memory to Lua string and passing the string to a function that outputs it ("print", "gg.alert", "gg.toast", etc) should be enough to achieve desired result in your case. Here is an example of function for reading bytes from memory and constructing string from them: function readBytesToString(address, length) local values = {} for i = 0, length - 1 do values[i] = {address = address + i, flags = gg.TYPE_BYTE} end values = gg.getValues(values) local bytes = {} for i, v in ipairs(values) do bytes[i] = v.value & 0xFF end return string.char(table.unpack(bytes)) end Call it with address to read from as first argument and amount of bytes to read as second argument, for example: local startAddress = 0x12340000 local bytesToRead = 20 local result = readBytesToString(startAddress, bytesToRead) print(result)- 10 replies
-
1
-
Relevant explanation from StackOverflow answer: https://stackoverflow.com/a/6389082
-
It's because of fundamentals of how Lua is implemented. The implementations (official one and LuaJ) are single-threaded, therefore only one operation can be performed at a time. Nothing can be done about it without changing the implementation.
-
How does that achieve the goal though? Original "searchNumber" function needs to be called anyway to actually perform the search. When it's called, it will only return after the search is finished (successfully or by cancellation). So how would a coroutine do anything when Lua executes native (i.e. not Lua one) function?