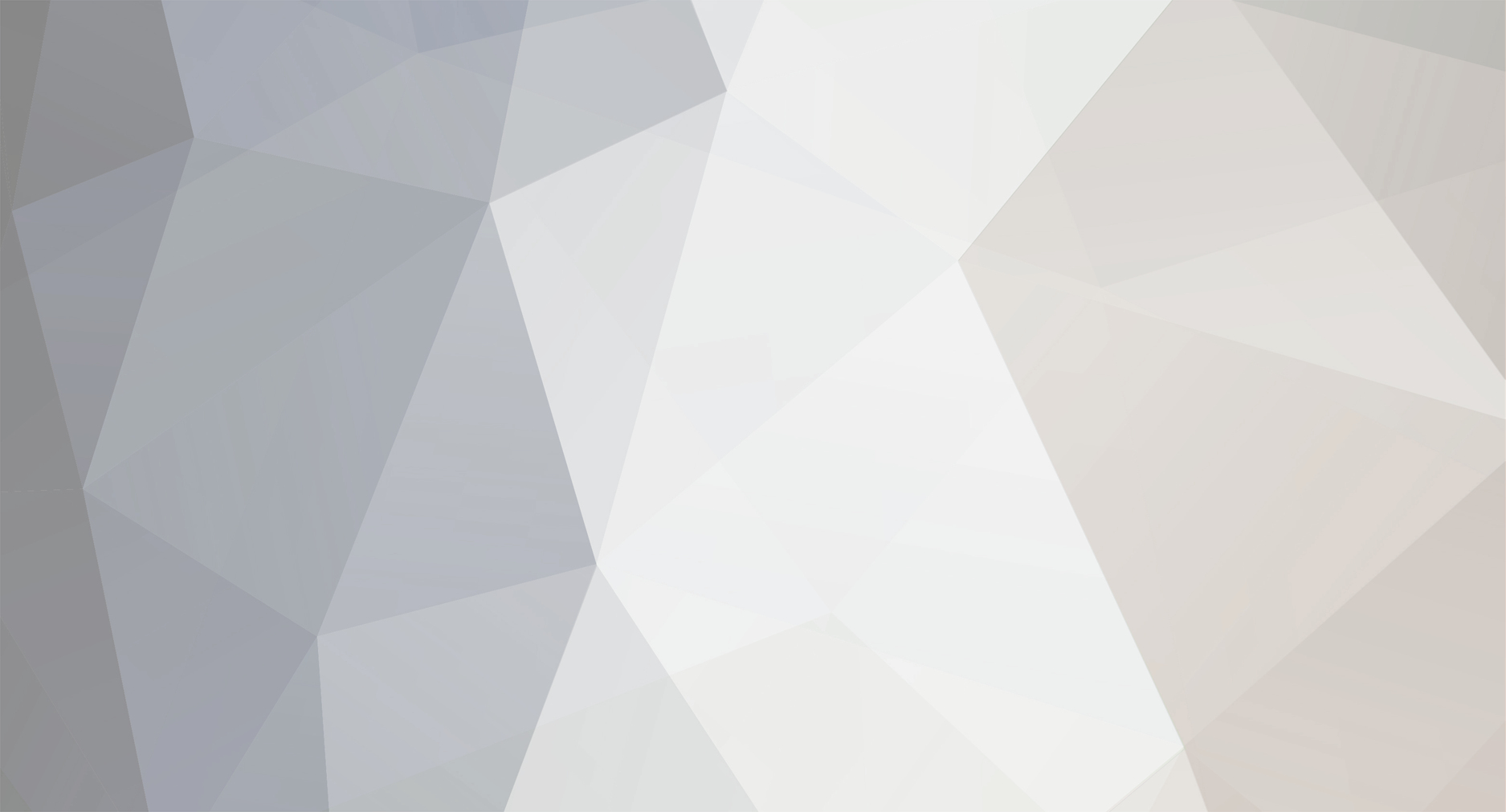
CmP
Contributor-
Posts
674 -
Joined
-
Last visited
-
Days Won
49
Content Type
Profiles
Forums
Downloads
Gallery
Everything posted by CmP
-
Yes, it should work. You can also move the call to "gg.removeListItems" outside the loop, since in the loop you construct table with list items to be removed.
-
Use bitwise operators. To check that address ends with hexadecimal digit C : v.address & 0xF == 0xC Similarly for D8: v.address & 0xFF == 0xD8
-
local function createPhaseDisplayer(phaseValues, progressSymbol, backgroundSymbol) local phasesCount = #phaseValues local currentPhase = 0 return function () currentPhase = currentPhase + 1 if currentPhase > phasesCount then os.exit() end local indicatorFirstPart = string.rep(progressSymbol, currentPhase) local indicatorSecondPart = string.rep(backgroundSymbol, phasesCount - currentPhase) local indicator = indicatorFirstPart .. indicatorSecondPart local phaseValue = phaseValues[currentPhase] local displayMessage = string.format("phase %d\nSymbol : %s\n%d", currentPhase, indicator, phaseValue) gg.toast(displayMessage, true) end end local phaseValues = {10, 20, 30, 40, 50} local symbol1 = "=" local symbol2 = "-" local displayPhase = createPhaseDisplayer(phaseValues, symbol1, symbol2) -- Testing all phases with one extra for i = 1, #phaseValues + 1 do displayPhase() end
-
Not very clear description, see yourself whether the following does what you want: local function setSpeedForSeconds(speed, seconds) gg.setSpeed(speed) gg.sleep(seconds * 1000) end while true do setSpeedForSeconds(1, 15) setSpeedForSeconds(5, 360) end
- 1 reply
-
1
-
From "Lua in GameGuardian" section of the documentation:
-
If this happens on emulator with binary translation (Bluestacks, LDPlayer, MEmu, etc) and instructions that are modified have been executed a bunch of times before modification, then this is expected. It's not a bug in GG, it writes memory successfully, behavior will be same regardless of which external tool is used to write memory (as long as method is same, see PTRACE_POKEDATA in ptrace man page). Possible workaround is to apply modification early on, before target instructions have been executed.
-
@MonkeySAN modify the code to detect process bitness without relying on "getTargetInfo" (since it's not guaranteed to be available), for example like the following: local ranges = gg.getRangesList() local highestAddress = ranges[#ranges]["end"] local is64 = (highestAddress >> 32) ~= 0
-
View File fastmem Performance-oriented library for reading and writing memory in scripts. Refer to "examples.lua" for usage basics. Main functions: - readMemory: reads array of bytes to string - readMemoryParts: reads array of bytes in parts - writeMemory: writes array of bytes GitHub: https://github.com/CmP-lt/fastmem Submitter CmP Submitted 03/15/2025 Category Templates
-
Version 1.0
118 downloads
Performance-oriented library for reading and writing memory in scripts. Refer to "examples.lua" for usage basics. Main functions: - readMemory: reads array of bytes to string - readMemoryParts: reads array of bytes in parts - writeMemory: writes array of bytes GitHub: https://github.com/CmP-lt/fastmem -
How to store that data in your code effectively depends on which interface to choose an item script needs to provide. If user input is name of item, then table item names as keys and item identifiers as values can work well. If user chooses option from list of possible items, then it can be table of tables with each inner table having name and value fields. Below is an example of tables for both of mentioned options: -- Option 1 local items = { ["dirt"] = 123, ["stone"] = 456 } -- Option 2 local items = { {name = "dirt", value = 123}, {name = "stone", value = 456} }
-
Doesn't and can't in principle, because Lua implementation in GG is in Java (based on Luaj).
- 1 reply
-
2
-
This indicates that value consists of one or more float values that correspond to units. You might be able to find float value corresponding to currently biggest unit used in particular instance of BigNumber, for that you can try range search (for example, if displayed value is 217.58C, then search "217.57~217.59" or maybe even "216.5~217.6", which should include the target value, but may also find many unrelated ones).
-
You can insert code for logging amount of items in saved list before and after fragments of code to see where it works not as expected: local items = gg.getListItems() print("Amount of items in saved list is " .. #items)
-
To remove items from saved list GG API has removeListItems function. Below is an example of how to use it to remove all items with value 0 from the list. local savedItems = gg.getListItems() local itemsForRemove = {} for i, v in ipairs(savedItems) do if v.value == 0 then itemsForRemove[#itemsForRemove + 1] = v end end gg.removeListItems(itemsForRemove)
-
Right, but if it is not done, it will happen automatically once the script completes. It's not related to the error that you got, it was because file can't be created in specified path, so "io.open" returns nil and then it is indexed with "write" key, which is the error from your screenshot. For example, you can try the following code and check contents of the file after running it: local path = "/sdcard/test.txt" -- Change to any other path to file that can be written io.open(path, "w+"):write("123")
-
-
-
The question is what you define as working. You asked for the code that modifies value(s) from saved list that are named "R" to "-2.0" 20 times in loop. The code above should do exactly that. Maybe that's not exactly what you need?
-
local savedValues = gg.getListItems() -- Enough to get items once if list won't change during execution of loop local targetValues = {} for i, v in ipairs(savedValues) do if v.name == "R" then targetValues[#targetValues + 1] = { address = v.address, flags = v.flags, value = "-2.0" } end end for i = 1, 20 do gg.setValues(targetValues) -- doesn't really make sense without delay end And, please, don't mention anyone in questions that are not directed to someone specifically.
-
Try hiding GG interface (gg.setVisible(false)) before setting values.
-
local function hack1() gg.toast("Hack 1") end local function hack2() gg.toast("Hack 2") end local function menu() gg.toast("Menu") end gg.setVisible(false) while not gg.isVisible() do gg.sleep(100) end hack1() gg.setVisible(false) while not gg.isVisible() do gg.sleep(100) end hack2() gg.setVisible(false) while true do if gg.isVisible() then menu() end gg.sleep(100) end
-
View File Embed libraries finder This script consists of a module (Lua code organized in certain way) for finding addresses of native libraries that are loaded directly from apk (which is the case when android:extractNativeLibs manifest attribute is set to "false") and several examples of how to use the module. Finding name of libraries in the script is implemented by checking soname of memory regions that are loaded from apk (including splits), contents of which resemble library in ELF format. Libraries that don't have soname specified won't be found by the script. Though, typically, main libraries of interest in games have soname specified, so the script should be applicable in most cases when libraries are loaded directly from apk. If a library has soname specified, but the script doesn't find it, generate debug log (example 3 from the file) from attempt to find the library and include it in the comment with report of the issue. Submitter CmP Submitted 07/26/2024 Category Templates
-
Version 1.0
389 downloads
This script consists of a module (Lua code organized in certain way) for finding addresses of native libraries that are loaded directly from apk (which is the case when android:extractNativeLibs manifest attribute is set to "false") and several examples of how to use the module. The script works only for 64-bit processes. Finding name of libraries in the script is implemented by checking soname of memory regions that are loaded from apk (including splits), contents of which resemble library in ELF format. Libraries that don't have soname specified won't be found by the script. Though, typically, main libraries of interest in games have soname specified, so the script should be applicable in most cases when libraries are loaded directly from apk. If a library has soname specified, but the script doesn't find it, generate debug log (example 3 from the file) from attempt to find the library and include it in the comment with report of the issue. -
View File Tagged pointers helper This script addresses GG not supporting tagged pointers natively by providing two features (going to pointer and searching pointers) that work for both regular and tagged pointers. Additionally, pointer search supports searching pointers for multiple targets at once. Script is used by selecting item(s) for desired operation in any of GG interface tabs and pressing "Sx" button to invoke script menu and choose the operation. Credits: - @BadCase - for method of searching for tagged pointers to multiple targets at once. Submitter CmP Submitted 07/06/2024 Category Tools
-
3